There may be times you want to add a watermark, or a bit of text, to an image programmatically. Here's how to do that with ImageSharp. As of the this writing, it requires a couple pre-release NuGet packages, but the ImageSharp libraries seem to work pretty well for at least basic use. Note that they do change the API while the libraries are in pre-release/beta, so the code below may not work by the time the libraries get to release versions, though hopefully any changes will be small.
Here are the NuGet packages you'll want to reference (you'll need to check the Include prerelease checkbox if doing this through the UI if you do this near the time of this article):
SixLabors.Fonts
SixLabors.ImageSharp
SixLabors.ImageSharp.Drawing
And here's some example code to load an image and draw some watermark text on it:
using SixLabors.Fonts;
using SixLabors.ImageSharp;
using SixLabors.ImageSharp.Drawing.Processing;
using SixLabors.ImageSharp.PixelFormats;
using SixLabors.ImageSharp.Processing;
const string text = "©adamrussell.com";
const float WatermarkPadding = 18f;
const string WatermarkFont = "Roboto";
const float WatermarkFontSize = 64f;
var image = await Image.LoadAsync("source-filename.jpg");
FontFamily fontFamily;
if (!SystemFonts.TryGet(WatermarkFont, out fontFamily))
throw new Exception($"Couldn't find font {WatermarkFont}");
var font = fontFamily.CreateFont(WatermarkFontSize, FontStyle.Regular);
var options = new TextOptions(font)
{
Dpi = 72,
KerningMode = KerningMode.Normal
};
var rect = TextMeasurer.Measure(text, options);
image.Mutate(x => x.DrawText(
text,
font,
new Color(Rgba32.ParseHex("#FFFFFFEE")),
new PointF(image.Width - rect.Width - WatermarkPadding,
image.Height - rect.Height - WatermarkPadding)));
await image.SaveAsJpegAsync("output-filename.jpg");
Some of this is a little contrived for example purposes; e.g., you might not want to draw such a large watermark on an image. The example draws text of a simple copyright notice in the bottom right corner of the image. It does this by measuring the text to be drawn, then applying padding against the image dimensions. One downside to the code above is that it uses a particular font family name, and the font needs to be installed on the system, otherwise the code will throw an exception. "Roboto"
from the example is free, and can be easily installed on Windows, macOS, or Linux (if you want to run the above code, you'll need to make sure Roboto is installed, or just change WatermarkFont
to something that's installed on your system).
Here's an example output image from a photo I took of a fiddler crab:
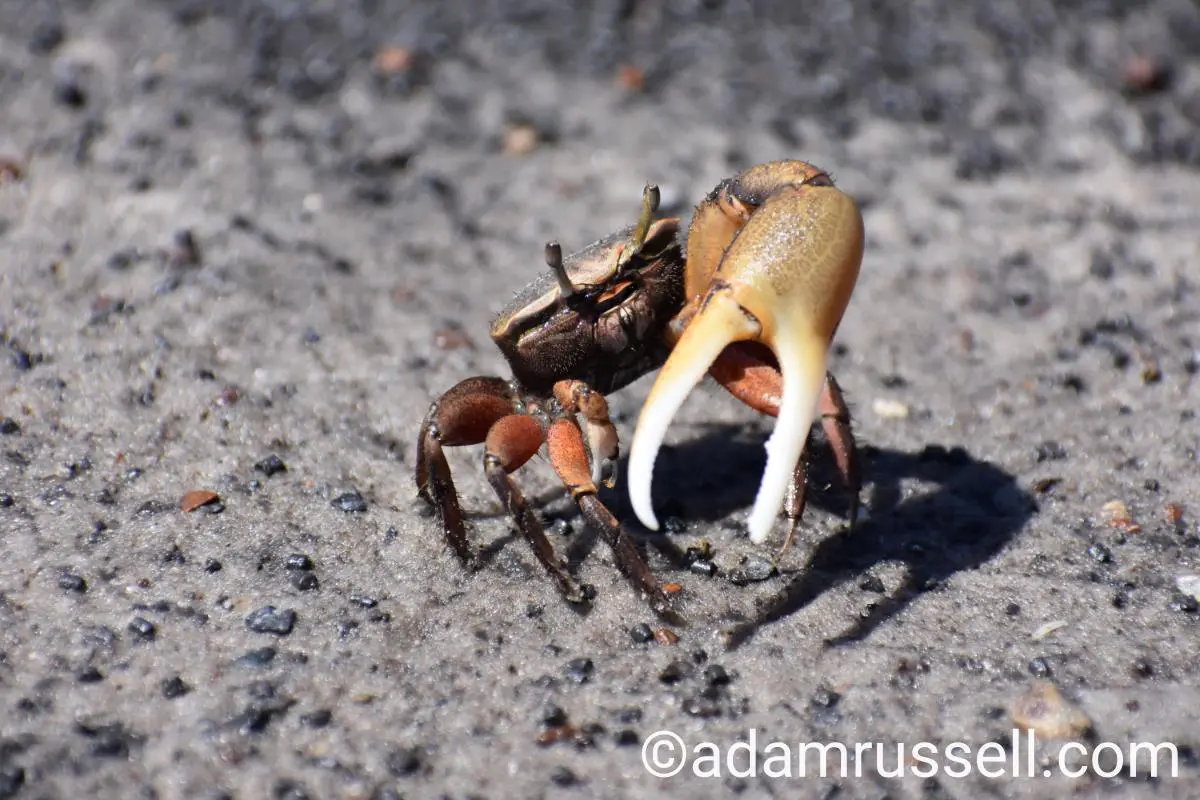